Addons
Addons allow you to extend the functionality of your Shop. With access to the powerful ProcessWire and Padloper APIs, the possibilities are limitless.
Here is a short demo of some example addons in action.
Types
There are currently two types of addons in Padloper; payment
and custom
addons. These are outlined below.
Payment Addons
Payment addons are payment gateways. Padloper ships with three core payment addons for PayPal, Stripe and Invoice payments. If you want to develop your own third-party payment addons, please see this documentation.
Custom Addons
These are any non-payment addons. They are referred to as custom addons
because you can customise them in any way you like. Custom addons are capable of doing many different things:
- They can be configurable, i.e., save settings
- They can process both ajax and non-ajax forms
- They can be used exclusively in the backend or can render markup in the frontend based on their saved configurations
- Etc.
Please see the documentation for custom addons if you wish to code your own.
Enabling Addons
The addons feature needs to be enabled in your shop's general settings before you can start activating and viewing your addons.
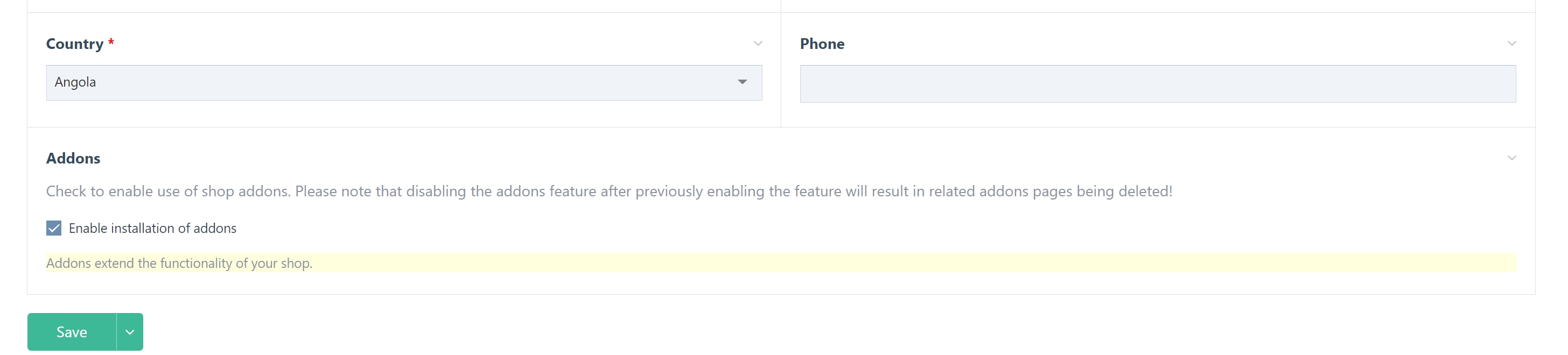
WARNING
If you disable the addons feature after previously enabling it, any addons previously activated will be deactivated and any configuration settings will be lost!
After enabling addons, a menu item named Addons
will appear under the Settings
menu. Click on that to navigate to the addons dashboard. If you have any addons, they will appear in this dashboard.
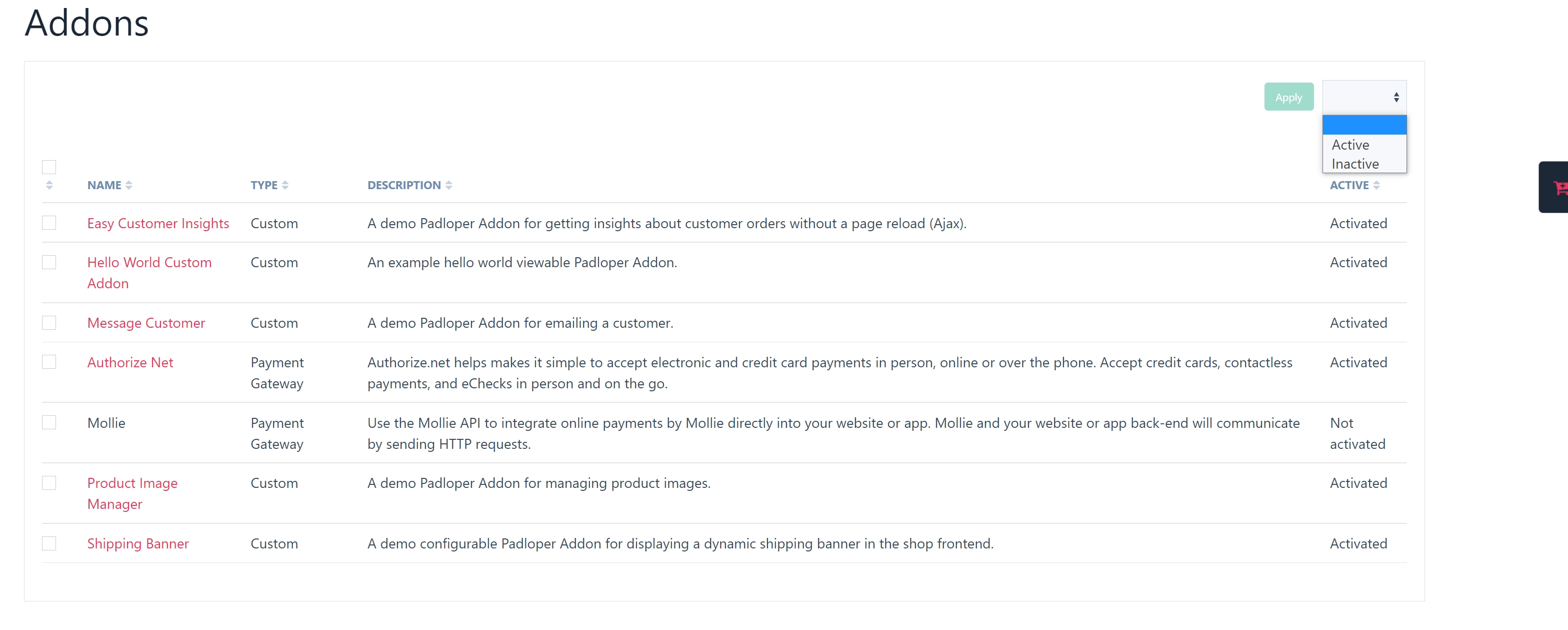
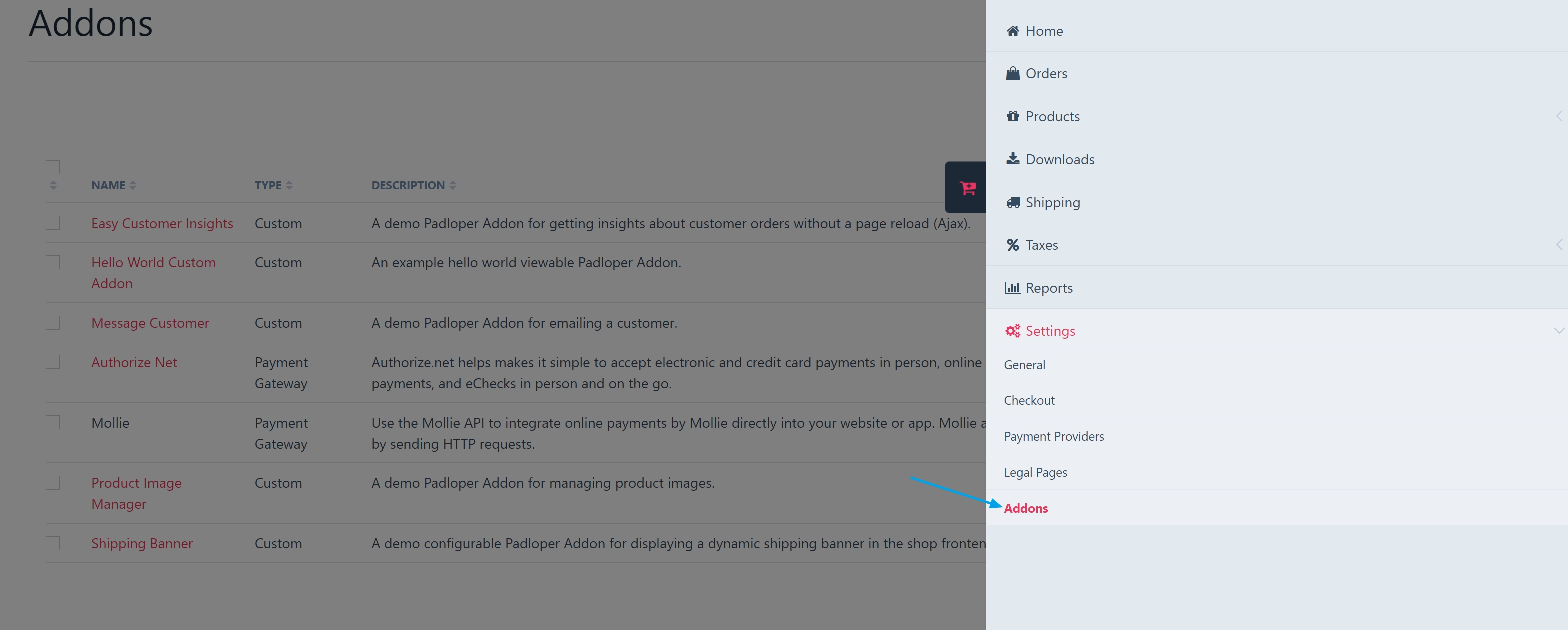
Addon Files
Your addon files should be stored in /site/templates/padloper/addons/
. Each addon should be in its own folder whose name should match the name of the addon class. For instance, an addon named MyCoolAddon
should be placed under /site/templates/padloper/addons/MyCoolAddon
.
Inside this folder there should be at least one file that contains the addon's PHP Class
. This file must have an identical name to its parent folder. The Class
inside this file must also have an identical name.
Hence, for your MyCoolAddon
, the path to the file with the Class should be MyCoolAddon.php
and the path will be /site/templates/padloper/addons/MyCoolAddon/MyCoolAddon.php
.
The addon Class
must implement the PadloperAddons Interface
. In this example, the name of the Class
would be MyCoolAddon
and it would implement the PadloperAddons Interface
.
The above rules allow Padloper to see your addons and load the required addon Class file.
WARNING
If your addons are not showing up in the addons dashboard list make sure they adhere to the above rules.
You can have as many addons as you want as long as they follow the above rules.
Activating / Deactivating Addons
In the addons dashboard, select the addons that you want to activate/deactivate and click apply.
WARNING
Deactivating a previously enabled addon will delete its configurations if it is a configurable addon.
View / Edit Addons
Only activated addons are viewable and editable. Click on the name of an activated addon to launch its view (and possibly, edit) page. Please note that for payment addons, this will navigate to its payment provider edit screen.
INFO
Activated Payment Addons will also be displayed in the payment providers.
Structure of Addons Files
How to structure addon files is up to the developer. A possible structure for a big addon could be:
/MyCoolAddon/
|-- MyCoolAddon.php
|-- my_cool_addon_partial.php
|-- MyCoolAddon.js
|-- MyCoolAddon.css
2
3
4
5
6
MyCoolAddon.php
This is the file with your addon class and is a required file.
my_cool_addon_partial.php
You can use this to store some of your addon markup, similar to /site/templates/_main.php
. You can load this file as a TemplateFile
from within MyCoolAddon.php
and pass variables to it . For example, as shown below.
private function renderPartial() {
// MyCoolAddon.php
$partialTemplate = new TemplateFile(__DIR__ . DIRECTORY_SEPARATOR . "my_cool_addon_partial.php");
// ---------------
// set properties for the template
// $partialTemplate->set('variable', 'someValue');
// --------
return $partialTemplate->render();
}
2
3
4
5
6
7
8
9
This helps to keep your MyCoolAddon.php
clean.
Additionally, you can use inline CSS
and/or JavaScript
inside your partial template
file.
MyCoolAddon.js
Use this to keep your JavaScript
separate from your markup, for instance, if the code is long.
If you experience loading order issues with respect to JavaScript
files, for instance if using third-party libraries, you will most likely want to call this file from your MyCoolAddon.php
instead of loading it from my_cool_addon_partial.php
. Hence, you can send the file to the browser by using ProcessWire's $config->scripts
as follows:
// MyCoolAddon.php
protected function render() {
$path = $this->wire('config')->urls->templates . "padloper/addons/MyCoolAddon/";
$this->wire->config->scripts->add("{$path}MyCoolAddon.js");
}
2
3
4
5
MyCoolAddon.css
Use this to keep your CSS
separate from your markup if you want to.
// MyCoolAddon.php
protected function render() {
$path = $this->wire('config')->urls->templates . "padloper/addons/MyCoolAddon/";
$this->wire->config->styles->add("{$path}MyCoolAddon.css");
}
2
3
4
5
Addons API
Padloper addons API allows you to retrieve your addon Class or saved configurations for use in the frontend of your shop. Please see its documentation here